Introduction
In the world of Object-Oriented Programming (OOP), two essential concepts stand out: Inheritance and Polymorphism. These concepts are the building blocks of code reusability, maintainability, and flexibility. In Python, they play a pivotal role, allowing developers to create efficient and modular applications. In this comprehensive guide, we’ll delve into the concepts of Inheritance and Polymorphism, exploring how they can empower your Python code.
Understanding Inheritance in Python
Inheritance is the mechanism by which a new class (child or subclass) can inherit attributes and methods from an existing class (parent or superclass). This fosters code reusability, enabling you to build upon an established blueprint without reinventing the wheel.
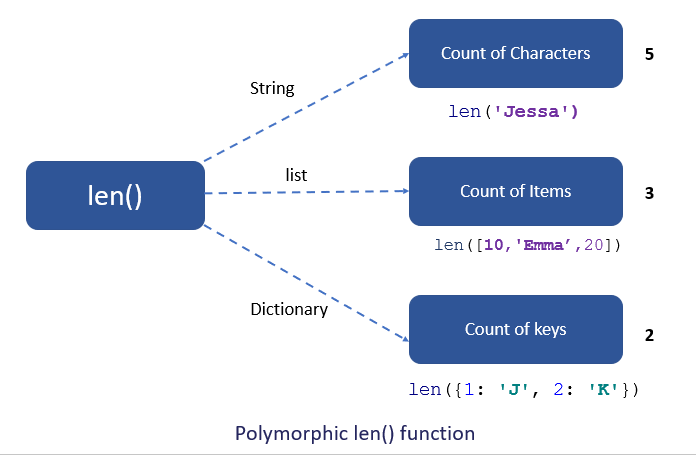
Key Inheritance Concepts:
- Superclass (Parent Class): The class whose attributes and methods are inherited is called the superclass or parent class.
- Subclass (Child Class): The class that inherits attributes and methods from the parent class is called the subclass or child class.
- Overriding: In Python, a subclass can provide its own implementation of a method from the parent class, replacing or “overriding” the inherited method. This allows customization and specialization.
Creating an Inheritance Hierarchy
To establish an inheritance relationship in Python:
- Define the Parent Class: Create the parent class with its attributes and methods.
- Define the Child Class: Create the child class, specifying the parent class in parentheses after the class name. This indicates that the child class inherits from the parent class.
- Override Methods (Optional): If necessary, you can override methods in the child class to provide custom behavior.
- Accessing Parent Methods: Within the child class, you can access methods and attributes from the parent class using the
super()
function.
Benefits of Inheritance
Inheritance brings several advantages:
- Code Reusability: Inherited attributes and methods save time and effort when building new classes.
- Modularity: Inheritance promotes code organization and modularity, making your codebase more manageable.
- Maintenance: Changes made to the parent class automatically affect all child classes, reducing redundancy and the chance of introducing bugs.
Understanding Polymorphism in Python
Polymorphism, derived from the Greek words “poly” (many) and “morph” (forms), refers to the ability of different classes to respond to the same method or function name in a way that is specific to their own class. In other words, it allows objects of different classes to be treated as objects of a common base class.
Key Polymorphism Concepts:
- Polymorphic Method: A method that can behave differently depending on the object calling it. In Python, polymorphism is achieved through method overriding.
- Base Class: The parent class that defines a polymorphic method is often referred to as the base class.
- Derived Classes: The child classes that implement their own versions of the polymorphic method are known as derived classes.
Implementing Polymorphism
To implement polymorphism in Python:
- Define the Base Class: Create a base class with a method that you intend to make polymorphic.
- Define Derived Classes: Create derived classes that inherit from the base class and override the polymorphic method. Each subclass provides its own unique implementation.
- Common Interface: Ensure that all derived classes adhere to a common method name and signature, allowing them to be used interchangeably.
Benefits of Polymorphism
Polymorphism offers several benefits:
- Flexibility: Different objects can respond to the same method, allowing for flexible code that can accommodate various data types.
- Code Reduction: Polymorphism reduces the need for complex conditional statements, leading to cleaner and more maintainable code.
- Enhanced Readability: Code that leverages polymorphism is often more readable and intuitive.
Conclusion
Inheritance and Polymorphism are essential concepts in Object-Oriented Programming, and mastering them can significantly enhance your Python code. Inheritance promotes code reusability, modularity, and maintenance, while Polymorphism brings flexibility and cleaner code. By understanding and implementing these concepts, you can build more efficient, maintainable, and flexible applications in Python. So, embrace the power of Inheritance and Polymorphism to take your Python programming skills to the next level.