Introduction
File handling is a fundamental aspect of programming, and in the world of Python, it is no different. Whether you’re working with data, configuration files, or text documents, Python provides a robust set of tools and techniques to make file handling efficient and straightforward. In this comprehensive guide, we’ll explore the ins and outs of file handling in Python, from opening and reading files to writing and manipulating data. Let’s dive into this essential skill that every Python developer should master.
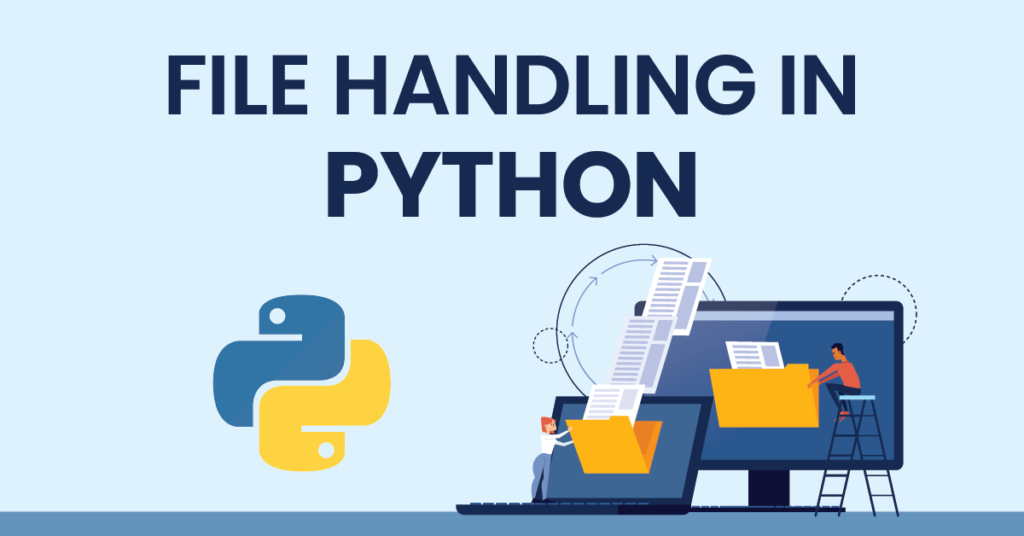
Understanding File Handling in Python
File handling in Python revolves around three key operations: reading, writing, and manipulating files. Python allows you to interact with various types of files, including text files, binary files, and more. Here’s a breakdown of each operation:
- Reading Files: Reading a file involves opening an existing file and extracting its content. You can read text or binary data, line by line or in one go.
- Writing Files: Writing to a file allows you to create new files, append data to existing files, or completely overwrite the contents of a file.
- Manipulating Files: Python also provides tools to manipulate files, such as renaming, moving, and deleting them.
Let’s explore these operations in detail.
Reading Files in Python
To read a file in Python, you typically follow these steps:
- Opening the File: Use the
open()
function to open a file. Specify the file’s path and the mode (e.g., ‘r’ for reading). - Reading Data: Once the file is open, you can read data using methods like
read()
,readline()
, or by iterating through the file object. - Closing the File: It’s essential to close the file using the
close()
method when you’re done reading to free up system resources.
Writing Files in Python
To write to a file in Python, follow these steps:
- Opening the File: Use the
open()
function with a specified mode (‘w’ for writing, ‘a’ for appending, etc.) and provide the file path. - Writing Data: Write data to the file using methods like
write()
orwritelines()
. - Closing the File: As with reading, it’s important to close the file when you’ve finished writing.
File Handling Best Practices
Here are some best practices to ensure efficient and error-free file handling in Python:
- Use ‘with’ Statements: Using the
with
statement ensures that the file is properly closed after the block is executed, even if an error occurs. - Error Handling: Always implement error handling to deal with potential issues when working with files, such as file not found or permission errors.
- File Paths: Be mindful of file paths, especially when working with different operating systems. Use the
os.path
module for path manipulation to ensure portability. - File Modes: Understand the different file modes (e.g., ‘r’, ‘w’, ‘a’, ‘b’) and select the appropriate mode for your task.
- File Encoding: When working with text files, specify the encoding, such as ‘utf-8’, to avoid potential character encoding issues.
Conclusion
Mastering file handling is an essential skill for any Python developer. Whether you are reading data from a file, creating log files, or parsing configuration files, Python’s file handling capabilities provide a powerful and flexible solution. By understanding the basic principles of reading, writing, and manipulating files, and following best practices, you can ensure your code is both efficient and error-resistant. So, go ahead and explore the world of Python file handling to unlock new possibilities and streamline your data-driven projects.