Introduction
Object-Oriented Programming (OOP) is a fundamental paradigm in computer science, providing a structured and efficient way to model real-world entities and their interactions in software. In Python, OOP is not just a feature; it’s a core concept that empowers developers to build modular, maintainable, and scalable applications. In this comprehensive guide, we’ll explore the world of OOP in Python, focusing on the cornerstone of this paradigm: classes and objects.
Understanding Classes and Objects
In OOP, a class is a blueprint for creating objects, which are instances of that class. Think of a class as a cookie cutter, and an object as a freshly baked cookie. The class defines the structure and behavior, while the object is the actual instance that you can work with.
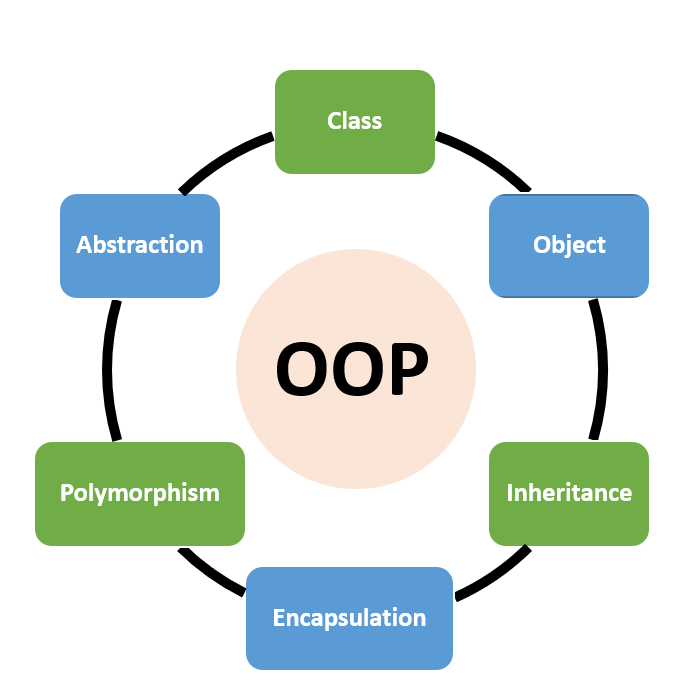
Key Concepts:
- Class: A class is a user-defined data structure that represents a blueprint for creating objects. It defines attributes (variables) and methods (functions) that describe the behavior and characteristics of the objects.
- Object: An object is an instance of a class, created based on the class’s blueprint. It has its unique set of attributes and can perform actions as defined by the class’s methods.
Creating and Using Classes in Python
Here’s how you create and use classes in Python:
- Defining a Class: You define a class using the
class
keyword, followed by the class name (conventionally using CamelCase). Inside the class, you define attributes and methods. - Attributes: Attributes are variables that hold data. They represent the characteristics of the class. For example, a
Car
class may have attributes likemake
,model
, andyear
. - Methods: Methods are functions defined within the class. They define the behavior of the class and can manipulate its attributes. For instance, a
Car
class might have methods likestart_engine()
andaccelerate()
. - Creating Objects: You create objects (instances) of a class by calling the class as if it were a function. For example,
my_car = Car()
creates an instance of theCar
class. - Accessing Attributes and Methods: You can access attributes and methods of an object using the dot notation, like
my_car.make
ormy_car.start_engine()
.
Encapsulation, Inheritance, and Polymorphism
OOP also introduces essential concepts like encapsulation, inheritance, and polymorphism:
- Encapsulation: This is the practice of bundling data (attributes) and the methods that operate on that data (functions) into a single unit, i.e., a class. It helps in data hiding and maintaining code integrity.
- Inheritance: Inheritance allows you to create a new class (child class) by inheriting attributes and methods from an existing class (parent class). This promotes code reuse and enhances modularity.
- Polymorphism: Polymorphism is the ability of different classes to respond to the same method or function name in a way that is specific to their own class. This concept simplifies code and promotes flexibility.
Benefits of Object-Oriented Programming
OOP brings numerous advantages to software development:
- Modularity: Classes allow you to organize your code into manageable modules, enhancing code reusability and maintainability.
- Abstraction: You can hide complex implementation details behind a simple interface, making code more comprehensible.
- Encapsulation: Data hiding ensures data integrity and reduces the risk of unintended modifications.
- Inheritance: Promotes code reuse, reducing redundancy and facilitating changes to the parent class.
- Polymorphism: Enhances flexibility and adaptability by allowing different objects to respond differently to the same method call.
Conclusion
Object-Oriented Programming, with its core concepts of classes and objects, is a powerful approach to software development in Python. It enables developers to design structured, modular, and maintainable code. By understanding and implementing OOP principles, you can create flexible, scalable, and efficient applications that stand the test of time. So, embark on your journey to mastering OOP in Python and take your coding skills to the next level.