In the realm of programming, variables and data types form the bedrock upon which the entire structure of a language is built. JavaScript, being a dynamically-typed language, empowers developers with flexibility in handling data. In this blog, we’ll embark on a journey into the world of variables and data types in JavaScript, unraveling the fundamental concepts that serve as the building blocks for dynamic and expressive code.
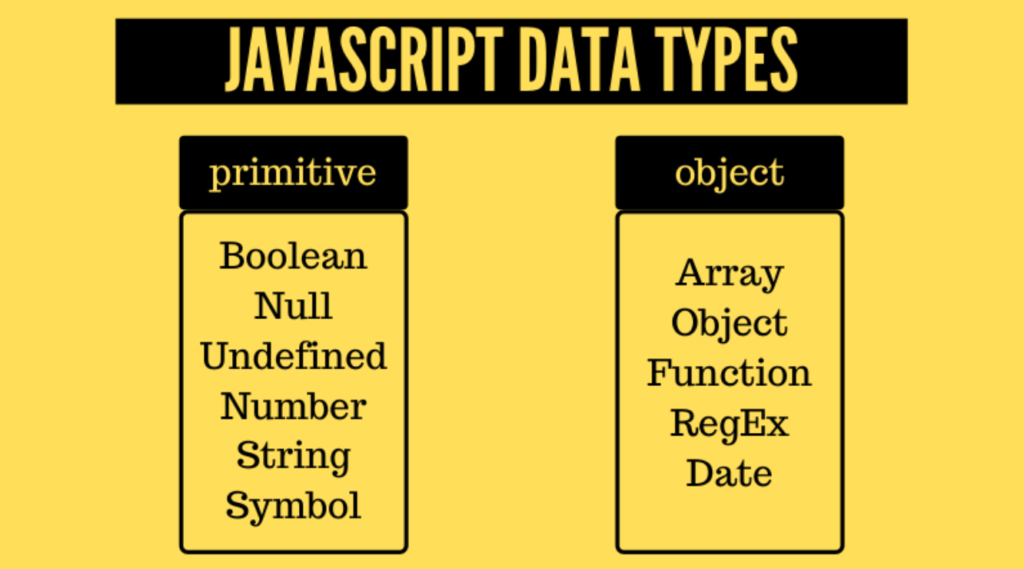
1. Variables: Containers of Information
At its core, a variable is a container for storing information. Unlike some statically-typed languages, JavaScript allows variables to hold different types of data throughout their lifecycle. The process of declaring a variable involves using the var
, let
, or const
keywords.
a. var
:
Historically used for variable declaration, var
has global scope or function scope, which can lead to unexpected behavior. It’s recommended to use let
and const
instead for more predictable scoping.
b. let
:
Introduced in ECMAScript 6 (ES6), let
allows block-scoping, limiting the variable’s accessibility to the block, statement, or expression in which it is defined.
c. const
:
Similar to let
in terms of block-scoping, const
declares a constant variable whose value cannot be reassigned. This is useful for values that shouldn’t change throughout the program.
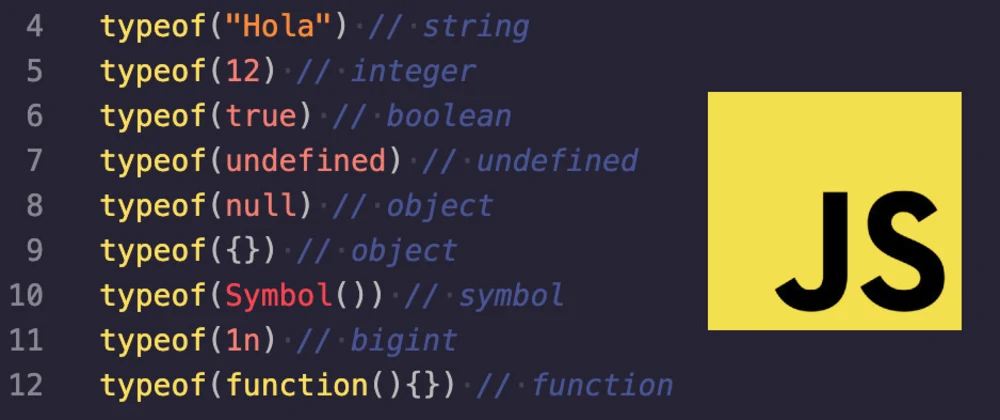
2. Data Types: Building Blocks of Information
JavaScript supports a variety of data types, each serving a specific purpose. Understanding these types is crucial for effective data manipulation and program execution.
a. Primitive Data Types:
- Number:
- Represents both integer and floating-point numbers.
- Example:
let age = 25;
- String:
- Represents a sequence of characters enclosed in single or double quotes.
- Example:
let name = 'John';
- Boolean:
- Represents a logical entity and can be either
true
orfalse
. - Example:
let isStudent = true;
- Null:
- Represents the intentional absence of any object value.
- Example:
let data = null;
- Undefined:
- Represents a variable that has been declared but not assigned a value.
- Example:
let undefinedVar;
- Symbol:
- Introduced in ES6, symbols are unique and immutable data types, often used to create unique object keys.
- Example:
const uniqueID = Symbol('id');
b. Object Data Type:
- Object:
- Represents a collection of key-value pairs, where values can be of any data type, including other objects.
- Example:
javascript let person = { name: 'Alice', age: 30, isStudent: false };
c. Special Data Types:
- BigInt:
- Introduced in ES2020, BigInt is used for representing arbitrary precision integers.
- Example:
const bigNumber = BigInt(9007199254740991);
3. Type Coercion and Conversion
JavaScript is known for its type coercion, where the interpreter automatically converts one data type to another during certain operations. Understanding how type coercion works is vital for preventing unexpected behavior in your code.
a. Explicit Conversion:
- Use functions like
parseInt()
,parseFloat()
,String()
, and others to explicitly convert values between types.
b. Implicit Conversion:
- JavaScript automatically converts types during operations, which can lead to unexpected results. Be mindful of type coercion when writing code.
Conclusion
As we wrap up our exploration of variables and data types in JavaScript, it’s essential to grasp the significance of these foundational concepts. Variables provide the means to store and manipulate data, while data types define the nature of that information. The dynamic and flexible nature of JavaScript shines through these concepts, allowing developers to build expressive and powerful applications. Armed with this knowledge, you’re now ready to dive deeper into the world of JavaScript programming, where variables and data types form the canvas upon which your code comes to life. Happy coding!