Introduction
Exception handling is a crucial aspect of any programming language, and in the realm of Python, it plays a central role in writing robust, error-free code. Exception handling allows developers to anticipate and gracefully manage unexpected errors and issues that may arise during program execution. In this comprehensive guide, we’ll explore the world of exception handling in Python, from understanding the basics to best practices for writing clean and reliable code.
Understanding Exceptions in Python
In Python, an exception is an event that can disrupt the normal flow of a program. These exceptions can be raised due to various reasons, including input validation, file operations, and more. Python provides a built-in mechanism to deal with exceptions, making it easier to address errors without crashing your program.
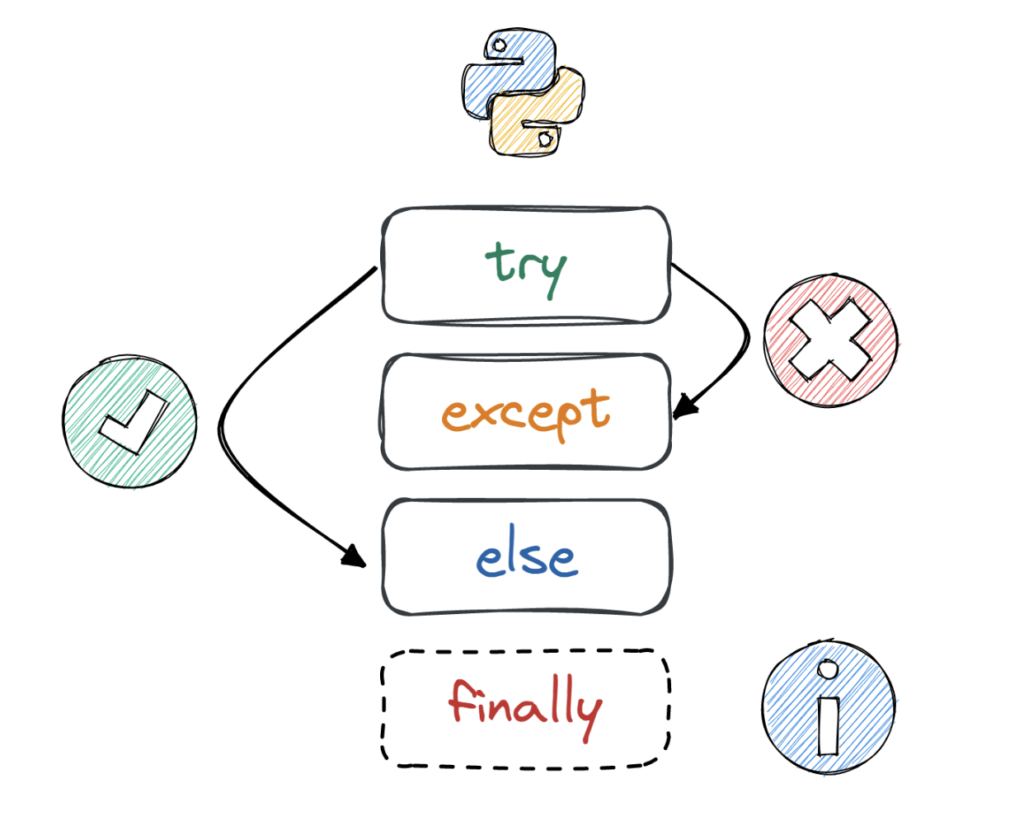
The Basic Exception Handling Structure
Python’s exception handling relies on a try-except block structure. Here’s how it works:
- Try Block: In this block, you place the code that might raise an exception. If an exception occurs within this block, Python will immediately jump to the appropriate exception handler.
- Except Block: This block contains the code that is executed when an exception is raised within the try block. You can have multiple except blocks to handle different types of exceptions.
- Finally Block (Optional): The finally block is executed regardless of whether an exception occurred or not. It’s often used for cleanup operations, like closing files.
Common Built-in Exceptions in Python
Python comes with a range of built-in exceptions to cover various types of errors. Some of the most common ones include:
TypeError
: Raised when an operation or function is applied to an object of an inappropriate type.ValueError
: Raised when a function receives an argument of the correct data type but with an inappropriate value.FileNotFoundError
: Raised when an attempt to open a file fails.ZeroDivisionError
: Raised when trying to divide by zero.IndexError
: Raised when trying to access an index that doesn’t exist in a list or sequence.
Best Practices in Exception Handling
- Specific Exception Handling: Catch specific exceptions rather than using a broad
except
clause. This allows you to handle different errors appropriately. - Don’t Use Bare
except
: Avoid usingexcept:
without specifying an exception type. It makes debugging difficult and may hide unexpected issues. - Use
finally
for Cleanup: When necessary, use afinally
block for cleanup operations, such as closing files or releasing resources. - Raising Custom Exceptions: You can define custom exceptions to make your code more descriptive and easier to debug.
- Logging: Utilize Python’s
logging
module to record error information, making it easier to diagnose issues in production.
Conclusion
Exception handling in Python is an indispensable skill for writing reliable and robust code. By understanding the basics of try-except blocks, knowing common exceptions, and implementing best practices, you can ensure that your programs handle errors gracefully and continue running even when unexpected issues arise. Exception handling is not just about managing errors; it’s also about creating code that is more resilient and easier to maintain. So, embrace Python’s exception handling capabilities to take your coding skills to the next level and deliver high-quality software solutions.