In the tapestry of JavaScript programming, operators and expressions weave the threads of logic, allowing developers to perform actions, make decisions, and manipulate data. In this blog, we’ll embark on a journey to unravel the intricacies of operators and expressions in JavaScript, understanding how they enable us to create dynamic and efficient code.
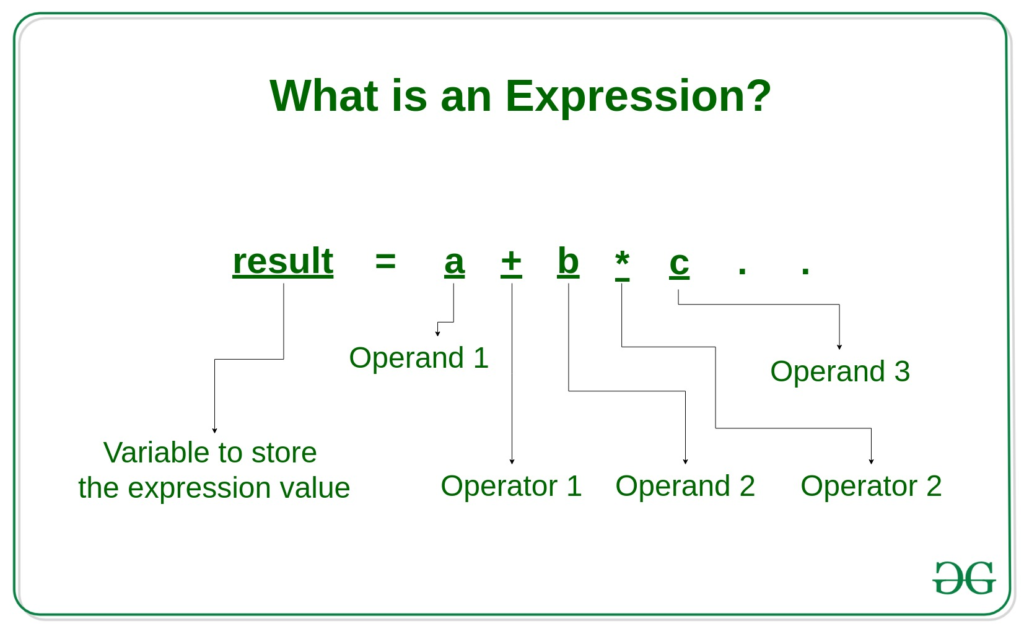
1. The Foundation: Operators
Operators in JavaScript are symbols that perform operations on operands. These operations can range from basic arithmetic to complex logical manipulations. Let’s explore the main categories of operators.
a. Arithmetic Operators:
- Addition (+): Adds two values.
- Subtraction (-): Subtracts the right operand from the left operand.
- Multiplication (*): Multiplies two values.
- Division (/): Divides the left operand by the right operand.
- Modulus (%): Returns the remainder of a division operation.
let result = 10 + 5; // result is 15
let remainder = 17 % 5; // remainder is 2
b. Comparison Operators:
- Equal (==): Returns true if the operands are equal.
- Not Equal (!=): Returns true if the operands are not equal.
- Strict Equal (===): Returns true if the operands are equal and of the same type.
- Strict Not Equal (!==): Returns true if the operands are not equal or not of the same type.
let isEqual = 5 == '5'; // isEqual is true
let isStrictEqual = 5 === '5'; // isStrictEqual is false
c. Logical Operators:
- AND (&&): Returns true if both operands are true.
- OR (||): Returns true if at least one operand is true.
- NOT (!): Returns true if the operand is false, and vice versa.
let isBothTrue = true && false; // isBothTrue is false
let isEitherTrue = true || false; // isEitherTrue is true
let isNotTrue = !true; // isNotTrue is false
d. Assignment Operators:
- Assignment (=): Assigns a value to a variable.
- Addition Assignment (+=): Adds the right operand to the left operand and assigns the result to the left operand.
- Subtraction Assignment (-=): Subtracts the right operand from the left operand and assigns the result to the left operand.
let x = 5;
x += 3; // x is now 8
x -= 2; // x is now 6
2. Crafting Logic: Expressions
Expressions are combinations of values, variables, and operators that can be evaluated to a single value. They form the building blocks of logic in JavaScript.
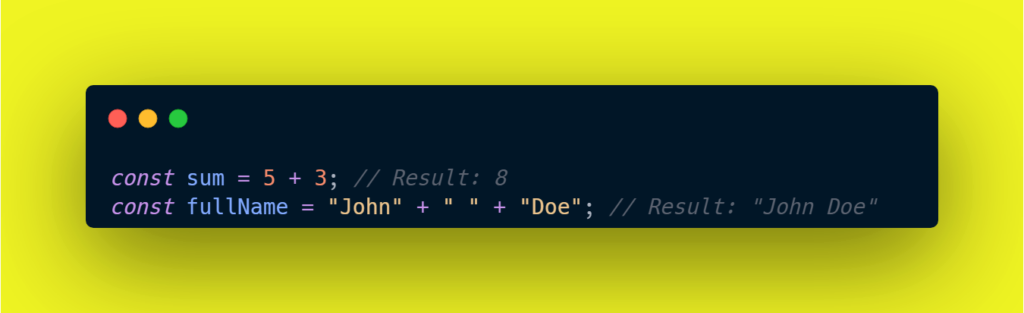
a. Arithmetic Expressions:
- Combining variables and values using arithmetic operators.
let total = (3 * x) + (2 * y);
b. Comparison Expressions:
- Using comparison operators to evaluate conditions.
let isGreater = x > y; // isGreater is true if x is greater than y
c. Logical Expressions:
- Crafting complex conditions using logical operators.
let isValid = (age >= 18) && (hasLicense || hasID);
d. Conditional (Ternary) Operator:
- A concise way to write conditional statements.
let status = (isOnline) ? 'Online' : 'Offline';
3. Operator Precedence and Associativity
Understanding operator precedence is crucial to deciphering complex expressions. It defines the order in which operations are performed. Parentheses can be used to override default precedence.
let result = 2 + 3 * 4; // result is 14, not 20
let overriddenResult = (2 + 3) * 4; // overriddenResult is 20
Conclusion
Operators and expressions form the backbone of JavaScript, enabling developers to craft logical and efficient code. Mastery of these concepts empowers you to manipulate data, make informed decisions, and express complex conditions concisely. As you continue your journey in JavaScript, these tools will prove invaluable in creating dynamic and responsive applications. So, dive into the world of operators and expressions, and let the logic flow through your code! Happy coding!